The four pillars of object-oriented programming are Abstraction, Encapsulation, Inheritance, and Polymorphism. This article explains the meaning of each.
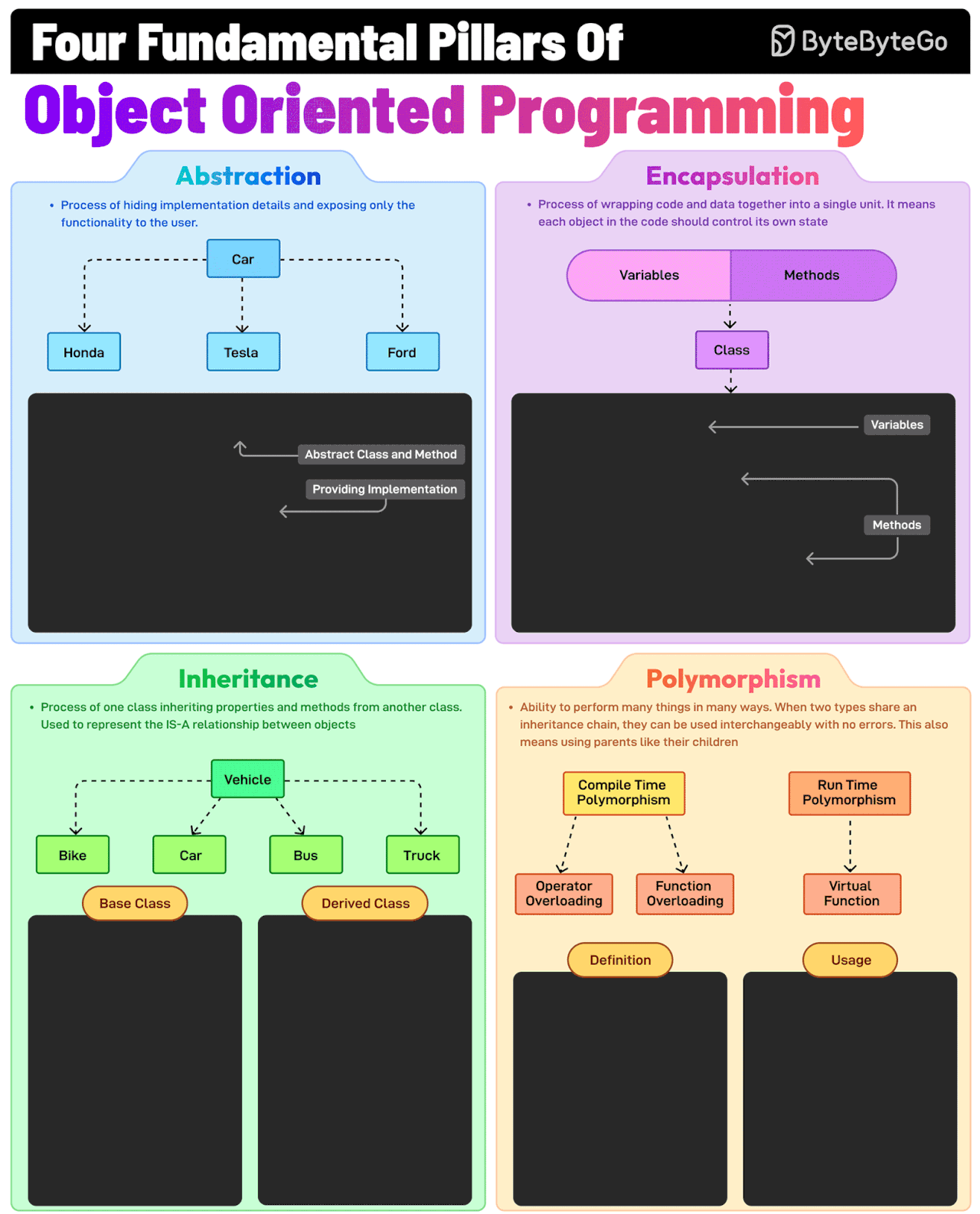
Abstraction is a fundamental concept in object-oriented programming that focuses on hiding complex implementation details while exposing only the essential features of an object.In programming terms, abstraction is implemented through abstract classes and interfaces. These provide a blueprint for what an object should do without specifying how it should do it.
Abstraction as a fundamental concept in object-oriented programming it focuses on hiding complex implementation details while exposing only the essential features of an object.
Think of a music streaming service – users only need to know how to play, pause, and select songs, not how the audio is decoded or streamed.
In programming terms, abstraction is implemented through abstract classes and interfaces. These provide a blueprint for what an object should do without specifying how it should do it.
Consider this example:
abstract class PaymentProcessor { abstract boolean validatePayment(); abstract void processPayment(); abstract String generateReceipt(); abstract void refundPayment(); } class CreditCardPayment extends PaymentProcessor { void processPayment() { // Specific credit card processing logic // Connecting to payment gateway // Handling encryption // Processing transaction } // Other implemented methods... } class CryptoCurrencyPayment extends PaymentProcessor { void processPayment() { // Specific cryptocurrency processing logic // Connecting to blockchain // Validating transactions // Managing wallet interactions } // Other implemented methods... }
Here, PaymentProcessor defines what every payment method should do without concerning itself with the specific implementation details. Each payment type can implement these methods according to its unique requirements.
Abstraction provides multiple advantages in software development:1. Complexity Management: By shielding users from intricate payment processing logic.2. Code Reusability: Allowing the reuse of an abstract payment structure for different payment methods.3. Security: Ensuring that sensitive payment processing details are kept hidden.4. Maintenance: Updates to payment implementations can be made without impacting the overall system.In real-world scenarios, abstraction is commonly employed in:- Payment gateways concealing transaction processing complexities- Authentication systems abstracting various login methods- File systems hiding storage and retrieval mechanisms
Effective practices for abstraction include:- Designing clean and intuitive interfaces- Abstracting only essential features- Maintaining consistent abstraction levels- Using clear and descriptive naming Convention by implementing proper abstraction, developers can build flexible, secure, and sustainable payment systems that can seamlessly adapt to emerging payment methods and technologies.
Encapsulation
Encapsulation is a core principle that bundles data and the methods that operate on that data within a single unit, restricting direct access to some of an object’s components. While abstraction focuses on hiding complexity, encapsulation focuses on data hiding and access control.
In programming terms, encapsulation is implemented through access modifiers and getter/setter methods. Consider this example building on our payment processor:
class CreditCardPayment extends PaymentProcessor { private String cardNumber; private String cardHolderName; private Date expiryDate; private int cvv; private TransactionStatus status; private List<PaymentTransaction> transactions; public void setCardDetails(String maskedCardNumber, String name) { this.cardNumber = encryptCardNumber(maskedCardNumber); this.cardHolderName = name; } public String getCardHolderName() { return this.cardHolderName; } public String getMaskedCardNumber() { return maskCardNumber(this.cardNumber); } // Private method for card encryption private String encryptCardNumber(String number) { return encryptedValue; } public TransactionStatus getTransactionStatus() { return this.status; } protected void updateTransactionHistory(PaymentTransaction transaction) { this.transactions.add(transaction); this.status = transaction.getStatus(); } }
Here, CreditCardPayment encapsulates sensitive payment data (cardNumber, cvv) and provides controlled access through methods. Direct access to critical payment information is prevented, ensuring security and data integrity.
Encapsulation provides various significant advantages in the context of payment systems.
Firstly, it ensures data security by safeguarding sensitive payment information from unauthorized access.
Additionally, controlled access mechanisms ensure that payment data can only be altered through verified methods, while data validation procedures guarantee that payment information is validated before processing.
Moreover, encapsulation offers implementation freedom by allowing internal payment processing details to be modified without impacting external code.
Real-world applications of encapsulation in payment systems include safeguarding sensitive payment credentials, securely managing transaction states, controlling access to payment history, and maintaining audit trails.
Adhering to best practices, such as making payment-sensitive data private, offering public methods solely for necessary payment operations, implementing robust validation in payment processing methods, concealing encryption and security mechanisms, and utilizing clear method names that accurately represent payment operations, developers can devise secure payment systems that protect sensitive financial data and present a streamlined interface for payment processing.
Ultimately, this approach enhances the reliability, security, and compliance of the payment system with financial regulations.
Inheritance
Inheritance enables a class to inherit attributes and methods from another class, promoting code reuse and establishing a hierarchical relationship between classes.
Indeed, inheritance is a fundamental concept in object-oriented programming that facilitates the organization and structuring of code. Through inheritance, a subclass can inherit attributes and methods from a superclass, reducing redundancy and promoting code reuse. This streamlines the development process and helps ensures a clear hierarchical relationship between classes, where the subclass is a specialized version of the superclass.By allowing a subclass to inherit attributes and methods from a superclass, developers can establish a hierarchical structure that reflects the “is a” relationship between different entities in the system.By utilizing inheritance, developers can create more modular, scalable, and maintainable codebases. The superclass serves as a template for common attributes and behaviors shared among multiple subclasses, fostering consistency and efficiency in software development.Furthermore, inheritance enables polymorphism, allowing objects of different classes to be treated uniformly through superclass references. This enhances flexibility and extensibility in the code, making it easier to add new features and functionalities without impacting existing code.Overall, when you think of Code reusability and code organization,think inheritance. Inheritance is the structure to adopt.
class PaymentProcessor { protected double amount; protected String currency; protected String merchantId; protected TransactionStatus status; public void setPaymentDetails(double amount, String currency) { this.amount = amount; this.currency = currency; } protected boolean validateAmount() { return amount > 0; } public TransactionStatus getStatus() { return status; } } class CreditCardPayment extends PaymentProcessor { private String cardNumber; private String cvv; private boolean internationalPayment; @Override protected boolean validateAmount() { // First check parent validation boolean baseValidation = super.validateAmount(); // Add credit-specific validation return baseValidation && amount <= getCreditLimit(); } } class CryptoPayment extends PaymentProcessor { private String walletAddress; private String networkType; private double gasPrice; @Override protected boolean validateAmount() { // First check parent validation boolean baseValidation = super.validateAmount(); // Add crypto-specific validation return baseValidation && amount >= getMinimumTransactionAmount(); } } class BankTransferPayment extends PaymentProcessor { private String accountNumber; private String routingNumber; private String bankName; private TransferType transferType; @Override protected boolean validateAmount() { // First check parent validation boolean baseValidation = super.validateAmount(); // Add bank-specific validation return baseValidation && amount <= getDailyTransferLimit(); } }
Key benefits of inheritance in this context
- Code Reuse: Common payment functionality is defined once in PaymentProcessor
- Extensibility: New payment methods can easily be added by extending PaymentProcessor
- Maintenance: Changes to core payment logic only need to be made in one place
- Polymorphic Behavior: Different payment types can provide their own implementations of common methods
Best practices for inheritance
- Follow “is-a” relationship (CreditCardPayment is a PaymentProcessor)
- Keep inheritance hierarchies shallow
- Use method overriding appropriately
- Maintain LSP (Liskov Substitution Principle)
- Don’t override methods just to throw exceptions
Real-world applications:
- Different payment providers with common base functionality
- Various payment validation rules
- Specialized payment processing requirements- Different fee structures for payment types
Polymorphism
Polymorphism is a fundamental concept in object-oriented programming where objects of different classes can be treated as objects of a common base class. This allows for greater flexibility and reusability in software design. By leveraging polymorphism, you can write code that is more generic and can operate on a variety of different object types without needing to know their specific implementations.One of the key benefits of polymorphism is that it simplifies complex systems by enabling the implementation of methods that can work with objects of multiple types without the need for explicit type checking. Furthermore, polymorphism promotes the principle of encapsulation, as it allows objects to hide their implementation details while exposing a common interface for interaction. This enables developers to write code that is more modular and easier to understand, leading to improved code quality and productivity
Continuing with our payment processor example:
class PaymentProcessor { protected double amount; protected String transactionId; public void processPayment() { if (validatePayment()) { initiatePayment(); notifyUser(); } } protected abstract boolean validatePayment(); protected abstract void initiatePayment(); protected abstract void calculateFees(); } class CreditCardPayment extends PaymentProcessor { @Override protected boolean validatePayment() { return validateCard() && checkCreditLimit(); } @Override protected void initiatePayment() { connectToCardNetwork(); processCardTransaction(); updateCardBalance(); } @Override protected void calculateFees() { // 2.5% + $0.30 for credit cards return amount * 0.025 + 0.30; } } class CryptoPayment extends PaymentProcessor { @Override protected boolean validatePayment() { return validateWalletAddress() && checkBlockchainNetwork(); } @Override protected void initiatePayment() { connectToBlockchain(); validateGasFees(); executeSmartContract(); } @Override protected void calculateFees() { // Variable gas fees for crypto return getCurrentGasFee() * estimateTransactionSize(); } } // Usage demonstrating polymorphismpublic class PaymentSystem { public void handlePayment(PaymentProcessor payment) { payment.processPayment(); // Calls appropriate implementation } public static void main(String[] args) { PaymentProcessor payment1 = new CreditCardPayment(); PaymentProcessor payment2 = new CryptoPayment(); // Same method call, different behaviors handlePayment(payment1); // Processes credit card payment handlePayment(payment2); // Processes crypto payment } }
Key aspects of polymorphism demonstrated:
- Runtime Polymorphism: Methods behave differently based on the actual object type
- Method Overriding: Each payment type implements its own version of common methods
- Interface Consistency: All payment types can be treated as PaymentProcessor objects
- Type Flexibility: Payment processing system works with any payment type
Benefits of polymorphism:
- Flexibility: New payment types can be added without changing existing code
- Maintainability: Common interface makes code easier to understand and maintain
- Extensibility: System can handle new payment methods through the same interface
- Code Organization: Related behaviors are grouped by payment type
Best practices:
- Design clear and cohesive interfaces
- Use meaningful method names that work for all implementations
- Maintain consistent behavior patterns across different implementations
- Document expected behavior for overridden methods
- Follow LSP (Liskov Substitution Principle)
Through proper encapsulation, developers can create secure payment systems that protect sensitive financial data while providing a clean interface for payment processing. This makes the payment system more reliable, secure, and compliant with financial regulations.
With proper inheritance, developers can create flexible payment systems that are easy to extend with new payment methods while maintaining consistent core functionality across all payment types. This approach reduces code duplication and ensures consistent behavior across different payment implementations.
Using polymorphism, developers can create flexible payment systems that handle multiple payment types uniformly while allowing each type to implement its specific processing logic. This creates maintainable, extensible code that can easily adapt to new payment methods and requirements.